MAP API
AIzaSyCM05666DmssJBC9g_sstrp09GDXLHv7sQ
Disable JetPack CSS – PHP
/* Disable Jetpack CSS */
add_filter( 'jetpack_implode_frontend_css', '__return_false', 99 );
Current Month Script – PHP
// Register the [month] shortcode
add_shortcode('month', 'custom_month_shortcode');
// Callback function for the [month] shortcode
function custom_month_shortcode() {
// Get the current month (e.g., "June")
$current_month = date('F');
// Return the current month
return $current_month;
}
Rank Math [year] Script – PHP
// Create Year shortcode to replace with current year.
add_shortcode( 'year', 'current_year' );
function current_year() {
$year = date( 'Y' );
return $year;
}
// To allow Rank Math Frontend title to parse shortcode.
add_filter(
'rank_math/frontend/title',
function ( $title ) {
return do_shortcode( $title );}
);
// Activate shortcode function in Post Title.
add_filter( 'the_title', 'do_shortcode' );
Prettify Blog Content
.content-wrapper p+:is(h2, h3, h4, h5, h6){
margin—top: 2em;}
Heading Overlay
.heading-overlay {
display: inline-block;
position: relative;
height: 5px;
border-radius: 30px;
background-color: #02A388;
width: 90px;
margin-top: 0px;
margin-bottom: 16px;
overflow: hidden;
}
.heading-overlay:after {
content: '';
position: absolute;
left: 0;
top: -1.1px;
height: 7px;
width: 8px;
background-color: #fff;
-webkit-animation-duration: 3s;
animation-duration: 3s;
-webkit-animation-timing-function: linear;
animation-timing-function: linear;
-webkit-animation-iteration-count: infinite;
animation-iteration-count: infinite;
-webkit-animation-name: MOVE-BG;
animation-name: MOVE-BG;
}
@keyframes MOVE-BG {
from {
-webkit-transform: translateX(0);
transform: translateX(0)
}
to {
-webkit-transform: translateX(88px);
transform: translateX(88px)
}
}
Animated Line
.animated-line {
position: relative;
display: inline-block;
height: 3px;
border-radius: 30px;
background-color: #0089d9;
width: 100px;
margin-top: 0;
margin-bottom: 14px;
overflow: hidden;
}
.animated-line:before,
.animated-line:after {
content: '';
position: absolute;
top: 0;
height: 3px;
width: 10px;
background-color: #fff;
border-radius: 30px;
}
.animated-line:before {
left: 0;
animation: move-dot-1 7s linear infinite;
}
.animated-line:after {
left: 20px;
animation: move-dot-2 7s linear infinite;
}
@keyframes move-dot-1 {
0%, 100% {
transform: translateX(0);
}
50% {
transform: translateX(80px);
}
}
@keyframes move-dot-2 {
0%, 100% {
transform: translateX(0);
}
50% {
transform: translateX(80px);
}
}
Perfecty White Colors
#perfecty-push-dialog-subscribe {
background-color: white !important;
color: #f77924 !important;
}
Centralize Snippet Box
#snippet-box {
margin: auto;
margin-bottom: 40px;
}
Bouncy Image

.bouncy-image{
animation: pbox1img 5s linear infinite;
animation-duration: 4s;
animation-timing-function: linear;
animation-delay: 0s;
animation-iteration-count: infinite;
animation-direction: normal;
animation-fill-mode: none;
animation-play-state: running;
animation-name: pbox5img;
}
@keyframes pbox5img {
0% {
transform: translateY(15px);
}
50% {
transform: translateY(-15px);
}
100% {
transform: translateY(15px);
}
}
Image Shine Effect
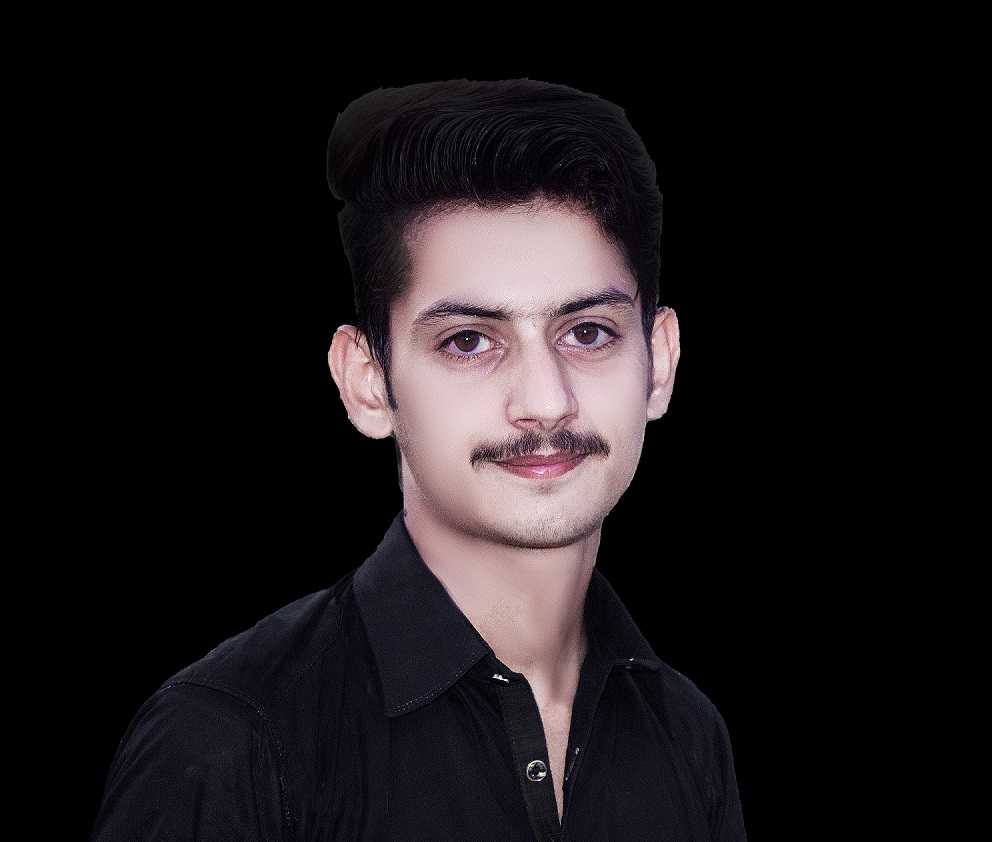
.shining-image {
position: relative;
overflow: hidden;
}
.shining-image::before {
content: '';
position: absolute;
top: 0;
left: -100%;
width: 100%;
height: 100%;
background: linear-gradient(to right, rgba(255, 255, 255, 0), rgba(255, 255, 255, 0.3), rgba(255, 255, 255, 0));
z-index: 1;
transform: skewX(-25deg);
transition: left 0.5s ease; /* Added ease for smoother transition */
}
.shining-image:hover::before {
animation: shineAnimation 1s forwards;
}
@keyframes shineAnimation {
to {
left: 120%;
}
}
APK Download Button Timer – HTML
<div id="countdown-message">Please wait while your download button appears in <span id="countdown">12</span> seconds...</div>
<a class="file-download-button" href="#">
<span class="gb-icon">
<svg viewBox="0 0 512 512" xmlns="http://www.w3.org/2000/svg"><path d="M288 32c0-17.7-14.3-32-32-32s-32 14.3-32 32V274.7l-73.4-73.4c-12.5-12.5-32.8-12.5-45.3 0s-12.5 32.8 0 45.3l128 128c12.5 12.5 32.8 12.5 45.3 0l128-128c12.5-12.5 12.5-32.8 0-45.3s-32.8-12.5-45.3 0L288 274.7V32zM64 352c-35.3 0-64 28.7-64 64v32c0 35.3 28.7 64 64 64H448c35.3 0 64-28.7 64-64V416c0-35.3-28.7-64-64-64H346.5l-45.3 45.3c-25 25-65.5 25-90.5 0L165.5 352H64zm368 56a24 24 0 1 1 0 48 24 24 0 1 1 0-48z"></path></svg>
</span>
<span class="file-button-text">Download APK File Now</span>
</a>
<script>
document.addEventListener("DOMContentLoaded", function() {
var countdownElement = document.getElementById("countdown");
var countdownMessage = document.getElementById("countdown-message");
var downloadButton = document.querySelector(".file-download-button"); // Selecting the download button by class
var counter = 20;
countdownElement.textContent = counter;
// Hide the download button initially
downloadButton.style.display = "none";
var countdownInterval = setInterval(function() {
counter--;
countdownElement.textContent = counter;
if (counter <= 0) {
clearInterval(countdownInterval);
countdownMessage.textContent = "Click below to start downloading...";
downloadButton.style.display = "inline-block"; // Show the download button
}
}, 1000);
});
</script>
APK Download Button Timer – CSS
.file-download-button {
margin-bottom: 24px;
border-radius: 10px;
padding: 14px 24px 14px 24px;
background: #056b88;
display: flex;
align-items: center;
justify-content: center;
text-decoration: none;
}
.file-download-button:hover {
background: #f5cc03;
}
.gb-icon svg {
fill: white;
width: 14px;
height: 14px;
margin-right: 8px;
}
.file-button-text {
color: white;
text-transform: uppercase;
}
Hover Slide Animation
This is dummy content
flhaflsqaflsdhflashdfls
.card-animation {
background: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease, box-shadow 0.3s ease;
}
.card-animation:hover {
transform: translateY(-10px);
box-shadow: 0 8px 16px rgba(0, 0, 0, 0.2);
}
Font Awesome CSS head tag
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.1/css/all.css">
Global Styling
/* Globel Styles */
:root {
--box-shadow: 0px 8px 15px -3px rgba(0,0,0,0.1);
--border-color: var(--global-color-10)
}
GP Primary Menu Hover Effect
.main-navigation li > a::after {
bottom: 10px;
content: "";
display: block;
height: 2px;
left: 0;
position: absolute;
background: var(--accent);
transition: width 0.2s ease 0s, left 0.1s ease 0s;
width: 0;
}
.main-navigation li > a:hover::after, .main-navigation li > a.active-nav::after {
width: calc(100% - 40px);
left: 50%;
transform: translatex(-50%);
bottom: 10px;
}
Image Block Settings
.entry-content img{
border-radius: 10px;
margin-bottom: 14px;
box-shadow: var(--box-shadow);
}
.entry-content figcaption {
font-size: .8rem;
margin-top: -10px;
text-align: center;
font-style: italic;
}
.no-shadow-image {
box-shadow: none !important;
}
.has-background {
border-radius: 10px;
box-shadow: var(--box-shadow);
}
Default Table Block Settings
/* TABLE BLOCK */
table {
box-shadow: var(--box-shadow);
}
/* Table head */
.wp-block-table thead {
text-align: left;
font-size: 0.9em;
border: 1px solid;
color: var(--contrast-2);
}
/* Table Rows - All rows */
.wp-block-table tbody tr {
font-size: 0.8em;
background-color: var(--global-color-10);
}
/* Table Rows - alternating row */
.wp-block-table tbody tr:nth-child(odd) {
background-color: var(--base-3);
}
/* Table body cells */
.wp-block-table tbody td {
border: 1px solid var(--border-color);
}
Sonic Customer BLOCK
/* Sonic Customer Service Box */
.sonic-info-box {
position: relative;
clear: both;
margin: 2.3rem 0rem 1.7rem 0rem;
border: solid .12rem var(--global-color-9);
border-radius: 10px;
padding: 1.875rem 1.3625rem;
padding-bottom: 0px;
width: 100%;
}
.sonic-info-box h2 {
display: table;
position: absolute;
transform: translate(-.75rem,-3.2rem);
padding: 0 .75rem;
line-height: 1.125;
font-weight: 400;
color: var(--global-color-9);
background-color: var(--base-3);
}
.sonic-info-box ul {
margin-left: 24px;
font-size: 1rem;
}
@media (max-width: 768px) {
.sonic-info-box ul {
font-size: 0.9rem;
}}
@media (max-width: 768px) {
.sonic-info-box h2 {
transform: translate(-.75rem,-3.6rem);
}}
Sticky Sidebar
@media (min-width: 768px) {
.sticky-container > .gb-inside-container,.sticky-container {
position: sticky;
top: 80px;
}
#right-sidebar .inside-right-sidebar {
height: 100%;
}
}
Disable Sidebar For Mobile
/* Disbale Sidebar For Mobile Devices */
@media(max-width: 768px) {
#right-sidebar, #left-sidebar {
display: none;
}
}
Responsive YouTube
/* Making YouTube Videos Responsive */
.iframe-container{
position: relative;
width: 100%;
padding-bottom: 56.25%;
height: 0;
margin-bottom: 24px;
}
.iframe-container iframe{
position: absolute;
top:0;
left: 0;
border-radius: 10px;
width: 100%;
height: 100%;
box-shadow: 0px 0px 10px 0px rgb(223 223 223), 0 0 40px rgb(50 0 140 / 0%) inset;
}
GP Author Box – GP BLOCK HTML
<!-- wp:generateblocks/container {"uniqueId":"f0f479ea","isDynamic":true,"blockVersion":4,"spacing":{"paddingBottom":"","paddingTop":"","paddingLeft":"","paddingRight":""},"className":"author-box"} -->
<!-- wp:generateblocks/grid {"uniqueId":"476eacc0","columns":2,"isDynamic":true,"blockVersion":3} -->
<!-- wp:generateblocks/container {"uniqueId":"0aa5d344","isGrid":true,"gridId":"476eacc0","isDynamic":true,"blockVersion":4,"sizing":{"width":"20%","widthMobile":"100%"}} -->
<!-- wp:generateblocks/image {"uniqueId":"4e23451e","mediaId":151,"sizeSlug":"full","width":"100px","height":"100px","alignment":"center","blockVersion":2,"useDynamicData":true,"dynamicContentType":"author-avatar","className":"author-avatar"} /-->
<!-- /wp:generateblocks/container -->
<!-- wp:generateblocks/container {"uniqueId":"adbf4970","isGrid":true,"gridId":"476eacc0","isDynamic":true,"blockVersion":4,"sizing":{"width":"80%","widthMobile":"100%"},"className":"author-info"} -->
<!-- wp:generateblocks/headline {"uniqueId":"158d8201","element":"div","blockVersion":3,"typography":{"fontSize":"19px"},"useDynamicData":true,"dynamicContentType":"author-name","className":"author-title"} -->
<div class="gb-headline gb-headline-158d8201 gb-headline-text author-title">Usman Hussain</div>
<!-- /wp:generateblocks/headline -->
<!-- wp:generatepress/dynamic-content {"contentType":"author-description"} /-->
<!-- wp:generateblocks/button {"uniqueId":"7558b0a7","hasUrl":true,"target":true,"relNoFollow":true,"blockVersion":4,"display":"inline-flex","alignItems":"center","columnGap":"0.5em","sizing":{"width":"","height":"","maxWidth":"","maxHeight":""},"spacing":{"paddingTop":"9px","paddingRight":"9px","paddingBottom":"9px","paddingLeft":"9px","marginRight":"","marginTop":"14px","marginBottom":"14px"},"borders":{"borderTopLeftRadius":"10px","borderTopRightRadius":"10px","borderBottomLeftRadius":"10px","borderBottomRightRadius":"10px"},"backgroundColor":"var(\u002d\u002dglobal-color-9)","backgroundColorHover":"var(\u002d\u002daccent)","textColor":"#ffffff","textColorHover":"#ffffff","hasIcon":true,"removeText":true,"iconStyles":{"height":"0.9em","width":"0.9em"}} -->
<a class="gb-button gb-button-7558b0a7" href="https://twitter.com/AllenRock121" target="_blank" rel="nofollow noopener noreferrer"><span class="gb-icon"><svg aria-hidden="true" role="img" height="1em" width="1em" viewBox="0 0 512 512" xmlns="http://www.w3.org/2000/svg"><path fill="currentColor" d="M459.37 151.716c.325 4.548.325 9.097.325 13.645 0 138.72-105.583 298.558-298.558 298.558-59.452 0-114.68-17.219-161.137-47.106 8.447.974 16.568 1.299 25.34 1.299 49.055 0 94.213-16.568 130.274-44.832-46.132-.975-84.792-31.188-98.112-72.772 6.498.974 12.995 1.624 19.818 1.624 9.421 0 18.843-1.3 27.614-3.573-48.081-9.747-84.143-51.98-84.143-102.985v-1.299c13.969 7.797 30.214 12.67 47.431 13.319-28.264-18.843-46.781-51.005-46.781-87.391 0-19.492 5.197-37.36 14.294-52.954 51.655 63.675 129.3 105.258 216.365 109.807-1.624-7.797-2.599-15.918-2.599-24.04 0-57.828 46.782-104.934 104.934-104.934 30.213 0 57.502 12.67 76.67 33.137 23.715-4.548 46.456-13.32 66.599-25.34-7.798 24.366-24.366 44.833-46.132 57.827 21.117-2.273 41.584-8.122 60.426-16.243-14.292 20.791-32.161 39.308-52.628 54.253z"></path></svg></span></a>
<!-- /wp:generateblocks/button -->
<!-- /wp:generateblocks/container -->
<!-- /wp:generateblocks/grid -->
<!-- /wp:generateblocks/container -->
GP Author Box – CSS
/* Author Box Under Posts */
.author-box {
padding: 3%;
padding-bottom: 10px;
margin-top: 30px;
font-size: 0.9em;
background-color: var(--base-3);
display: -webkit-box;
display: -ms-flexbox;
display: flex;
-webkit-box-align: center;
-ms-flex-align: center;
align-items: center;
box-shadow: var(--box-shadow);
border-radius: 20px;
}
.author-box .author-avatar {
width: 110px;
height: auto;
border-radius: 100%;
margin-right: 30px;
}
.author-title {
margin-bottom: 0.1em;
font-weight: 600;
font-size:18px;
}
.author-description {
line-height: 1.6em;
font-size:16px;
}
@media (max-width: 768px) {
.author-box {
padding: 20px;
padding-bottom: 25px;
margin-top: 60px;
flex-direction: column;
text-align: center;
}
.author-box .author-avatar {
margin-right: 0;
margin-top: -60px;
margin-bottom: 10px;
}
.author-box .author-avatar img {
max-width: 100px;
}
.author-description {
margin-bottom: -0.1em;
}
.author-profiles {
display: flex;
justify-content: center
}
}
Basic Scrapping – Python
import requests
from bs4 import BeautifulSoup
# URL of the main page
url = 'https://www.luckymodapk.com/games/racing/'
# List to store all the titles
titles = []
# Function to extract titles from a given URL
def extract_titles(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# Find the <ul> element with class "clearfix list-one-ul"
ul_element = soup.find('ul', class_='clearfix list-one-ul')
if ul_element:
# Find all <li> elements within the <ul> element
li_elements = ul_element.find_all('li')
for li in li_elements:
# Find the <div> tag with class "list-one-box clearfix"
div_element = li.find('div', class_='list-one-box clearfix')
if div_element:
# Find the <h1> tag with class "list-one-h1" inside the <div> element and extract its text
title_element = div_element.find('h1', class_='list-one-h1')
if title_element:
title = title_element.text
titles.append(title)
# Extract titles from the main page
extract_titles(url)
# Extract titles from pagination pages (pages 2 to 40)
for page_num in range(2, 41):
pagination_url = f'https://www.luckymodapk.com/games/racing/{page_num}/'
extract_titles(pagination_url)
# Print the final list of titles
print(titles)
URLs nd Title From Google – Python
import requests
from bs4 import BeautifulSoup
# URL of the main page
url = 'https://www.google.com/search?lr=lang_en&tbo=p&tbm=bks&q=Prophet+Muhammad&tbs=,bkv:f&num=100'
# List to store all the titles and URLs
titles_urls = []
# Function to extract titles and URLs from a given URL
def extract_titles_urls(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# Find all div elements with class 'Yr5TG'
div_elements = soup.find_all('div', class_='Yr5TG')
for div_element in div_elements:
# Find the <a> tag inside the <span> tag
a_tag = div_element.find('span').find('a')
if a_tag:
title = a_tag.text
url = a_tag['href']
titles_urls.append({'title': title, 'url': url})
# Extract titles and URLs from the main page
extract_titles_urls(url)
# Print the final list of titles and URLs
for item in titles_urls:
print(f"Title: {item['title']}\nURL: {item['url']}\n")